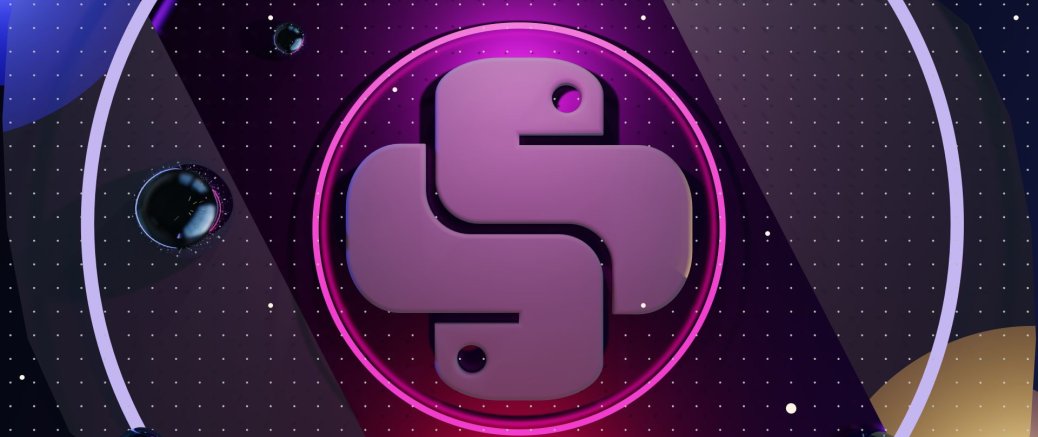
Introduction
Python is a popular high-level programming language that is widely used in various fields such as web development, data science, machine learning, artificial intelligence, and scientific computing. It was created in the late 1980s and is known for its simple syntax and readability, making it easy to learn and use. Python is an interpreted language, meaning that the code is executed line by line at runtime, which allows for rapid development and prototyping. It also has a vast collection of third-party libraries and frameworks that make it easy to accomplish complex tasks with minimal coding effort. Some of the popular libraries and frameworks include NumPy, Pandas, Django, Flask, TensorFlow, and PyTorch. Python is also known for its versatility and can be used for a wide range of applications, from building web applications to analyzing large datasets and creating machine learning models.
Python is a versatile programming language with many benefits, making it an excellent choice for both beginners and experienced programmers. One significant advantage of Python is its high demand in various industries, including web development, data science, machine learning, and artificial intelligence. Thus, learning Python can increase job opportunities and employability. Additionally, Python has a simple and intuitive syntax, making it easy to learn and use. Even beginners with no prior programming experience can start writing code quickly and efficiently.
Python also has a large and active community of developers who contribute to its development, provide support, and share knowledge through various forums, meetups, and online resources. This community is vital for learning Python and staying up-to-date with the latest developments and best practices.
Python is a very flexible programming language that can be used for a wide range of applications. It can be used to develop web applications, data analysis, automation, scientific computing, machine learning models, game development, and high-performance computing applications, among others. Python also has a vast collection of third-party libraries and frameworks that make it easy to accomplish complex tasks with minimal coding effort.
Python is an open-source programming language, meaning that the source code is freely available and can be modified and distributed without any restrictions. This openness makes it an excellent choice for developing open-source projects and collaborating with other developers. Overall, Python is an excellent programming language to learn, with many benefits for both personal and professional growth.
History of Python
Python was created in the late 1980s by Guido van Rossum, a Dutch programmer who wanted to create a language that was easy to learn and use. The first version of Python, Python 0.9.0, was released in 1991. Over the years, Python has evolved and grown in popularity, becoming one of the most widely used programming languages in the world.
One significant event in the history of Python was the transition from Python 2 to Python 3. Python 2 was released in 2000, and it quickly gained popularity. However, as the language grew and evolved, it became apparent that Python 2 had some design flaws and limitations that needed to be addressed. In response, the Python community began work on Python 3, which was released in 2008. Python 3 was designed to be more efficient, secure, and robust than Python 2, and it included many new features and improvements.
The transition from Python 2 to Python 3 was not without challenges. One of the most significant issues was the lack of backward compatibility between the two versions. Python 3 made several changes to the language that were not compatible with Python 2, making it challenging to migrate existing codebases. However, over time, the Python community has made significant progress in supporting Python 3, and many developers have migrated to the new version. Python 3 represents a significant improvement over its predecessor and has become the standard version of Python. Moving forward, the Python community will undoubtedly continue to develop and improve the language, ensuring its continued relevance and popularity for years to come.
Ease of Learning and Use
Python is widely known for its simplicity and ease of use. It has a straightforward syntax that is easy to understand and learn, even for beginners who have no prior programming experience. One reason for this is that Python uses a clean, readable, and concise code format, which makes it easy to write, read, and maintain code. The use of whitespace and indentation to denote code blocks also makes Python code more readable and easier to understand, compared to other programming languages that use curly braces or other symbols for code blocks. Of course curly brace supporters may disagree.
Another feature that makes Python easy to learn is its extensive documentation and rich community resources. Python has a vast and active community of developers who contribute to its development, provide support, and share knowledge through various forums, meetups, and online resources. The official Python documentation is comprehensive and well-structured, providing detailed explanations, examples, and reference material. Furthermore, Python has an extensive library of third-party packages and frameworks that make it easy to accomplish complex tasks with minimal coding effort.
In addition, Python's simplicity and ease of use make it a popular choice for teaching programming to beginners. Many universities, schools, and online learning platforms use Python as a primary programming language for teaching computer science and other related disciplines. Python's readability and ease of use also make it an excellent choice for rapid prototyping and development, allowing developers to quickly build and test ideas without spending too much time on syntax and other low-level details.
Overall, Python's simplicity and ease of use make it a great choice for beginners and experienced programmers alike. Its straightforward syntax, extensive documentation, and active community resources make it easy to learn and use, while its versatility and performance make it suitable for a wide range of applications. Here are a few short examples of Python code:
Example 1: Hello World!
This is a classic example of a "Hello World!" program in Python. It simply prints the string "Hello, World!" to the console.
print("Hello, World!")
Example 2: Adding two numbers
This code adds two numbers, 5 and 10, and prints the result to the console.
a = 5
b = 10
c = a + b
print(c)
Example 3: Fibonacci Sequence
Here's a more advance example of Python code that calculates the Fibonacci sequence using a recursive function:
def fibonacci(n):
if n <= 1:
return n
else:
return fibonacci(n-1) + fibonacci(n-2)
# Calculate the first 10 numbers in the Fibonacci sequence
for i in range(10):
print(fibonacci(i))
This code is much more complex. It defines a recursive function fibonacci(n) that takes an integer n as input and returns the nth number in the Fibonacci sequence. The function uses the recursive formula fibonacci(n) = fibonacci(n-1) + fibonacci(n-2) to calculate the value of each number in the sequence. The code then uses a for loop to calculate and print the first 10 numbers in the Fibonacci sequence by calling the fibonacci() function for each value of i in the range 0 to 9.
Versatility
Python is a versatile programming language that can be used in a wide range of applications due to its extensive library of modules and frameworks. One reason for Python's versatility is that it supports various programming paradigms, including procedural, object-oriented, and functional programming. This means that developers can use Python to write code in different styles, depending on the application requirements.
Python is widely used in web development, where it is used to build server-side applications, APIs, and web frameworks. Some popular web frameworks in Python include Flask, Django, and Pyramid, which provide an easy and efficient way to build web applications.
In addition to web development, Python is also widely used in scientific computing, data analysis, and machine learning. Python has many powerful libraries, including NumPy, SciPy, Pandas, and Matplotlib, that provide efficient tools for numerical computations, data analysis, and visualization. Furthermore, Python is the language of choice for many machine learning and deep learning libraries, such as TensorFlow, Keras, and PyTorch, due to its simplicity and ease of use.
Moreover, Python is used in system administration and automation. It is a powerful scripting language that is used to automate repetitive tasks and system administration tasks, such as file manipulation, network programming, and system monitoring.
Python is also used in game development, desktop applications, and mobile applications. The Pygame library provides an easy and efficient way to build 2D games in Python. Furthermore, Python can be used to build cross-platform desktop applications using frameworks like Tkinter, PyQt, and wxPython. Also, Python can be used to develop mobile applications using frameworks such as Kivy and BeeWare.
Python's large standard library is one of its major strengths, providing a wide range of modules and functions for different applications. The standard library contains modules for file input/output, regular expressions, network programming, threading, and more. This vast collection of modules allows developers to quickly and easily build complex applications without having to write everything from scratch.
Furthermore, Python is compatible with other languages, which makes it easy to integrate with other tools and applications. For instance, Python can be used with C/C++ libraries through modules such as ctypes or Cython. This enables developers to leverage existing C/C++ codebases in their Python projects or to write performance-critical code in C/C++ and then call it from Python.
Python can also be used with databases such as MySQL, PostgreSQL, and Oracle, which makes it an excellent choice for building database-driven applications. Additionally, Python can be used with various web servers, such as Apache, Nginx, and Gunicorn, allowing developers to build robust web applications.
Python is often used for system administration tasks and can be integrated with tools like Ansible, Salt, and Fabric to automate system administration tasks across multiple servers – including monitoring, file manipulation and webscraping. Python can also be used for data analysis and visualization, where it can be integrated with tools like Jupyter Notebook and Apache Spark.
Finally, Python is also compatible with other scripting languages such as Perl and Ruby, which allows developers to mix and match different languages in their projects. This compatibility with other languages and tools makes Python a versatile language that can be used in a wide range of applications and scenarios.
These days the seems to be almost nothing that Python cannot do with its ability to leverage its large standard library and interface with multiple languages and frameworks. These features make Python a versatile language that can be used for various purposes and in different industries and applications.
High Demand for Python Developers
Python is one of the most in-demand programming languages in the job market today, and this is supported by various data and statistics. For instance, the Stack Overflow Developer Survey 2021 found that Python is the third most popular programming language, after JavaScript and HTML/CSS. The survey also revealed that Python is the most commonly used language for data analysis, machine learning, and scientific computing.
Furthermore, job market trends show that Python is highly sought after by employers. According to the 2021 Developer Survey by HackerRank, Python was the most in-demand programming language by companies in the United States, followed by Java and JavaScript. The survey also found that Python was the most preferred language for data science, machine learning, and artificial intelligence.
Another indicator of Python's demand in the job market is the number of job postings that require Python skills. According to data from Burning Glass Technologies, Python was the third most requested programming language in job postings in the United States in 2020, after Java and JavaScript. This trend is expected to continue in the coming years, as more companies seek to leverage Python's capabilities for data analysis, machine learning, and automation.
In addition, the average salary of Python developers is also a testament to its high demand in the job market. According to Payscale, the average salary of a Python developer in the United States is around $78,000 per year, which is higher than the average salary of software developers who use other programming languages.
It is clear that Learning Python can open up new job opportunities and increase earning potential in several ways. As mentioned earlier, Python is one of the most in-demand programming languages in the job market today. This means that learning Python can make you a more attractive candidate to employers who are looking for these skills.
Additionally, learning Python can help you specialize in a particular area of software development, which can lead to higher salaries. For instance, according to Payscale, the average salary for a machine learning engineer is around $112,000 per year, while the average salary for a data scientist is around $96,000 per year. These salaries are higher than the average salary for software developers who do not have these specializations.
Another great plus for Python programmers is the opportunity it can afford for freelance or contract work. Many companies and organizations are looking for Python developers to work on short-term projects, such as building web applications, automating tasks, or analyzing data. Freelancing or contracting can provide more flexibility and autonomy, as well as potentially higher earning potential.
Python is also recognized as being able to lead to opportunities for entrepreneurship. Python is widely used in the development of startups and other small businesses, as it allows for the rapid development of prototypes and minimum viable products. By learning Python, you can develop your own software products, start your own consulting business, or create other entrepreneurial ventures.
Whether you are a software developer looking to specialize in a particular area, a freelancer or contractor looking for short-term projects, an entrepreneur looking to start your own business, or just a hobbyist, learning Python can be a valuable skill to have.
Community Support
Python has a large and active community of developers who contribute to the language's development and support. The community is made up of developers from around the world who share their knowledge, collaborate on projects, and help each other out when they encounter problems.
One of the main ways the Python community provides support is through online forums and mailing lists. These forums and lists are a place where developers can ask questions, get help with problems, and share their own knowledge and experiences. Some of the most popular forums and mailing lists include the official Python mailing list, Stack Overflow, and Reddit's Python community.
In addition to forums and mailing lists, the Python community also provides support through social media. There are many active Python communities on platforms such as Twitter, LinkedIn, and Facebook, where developers can connect with each other, share resources, and ask for help. YouTube has many high quality Python classes you can watch for free as well.
Another way the Python community supports developers is through the development and maintenance of open-source libraries and frameworks. These libraries and frameworks provide pre-built code that developers can use to solve common problems and speed up their development process, and extend the capabilities of Python. Here are some examples:
-
NumPy: NumPy is a library for numerical computing in Python. It provides an array data structure that is more efficient and convenient for numerical computations than the built-in Python list. NumPy is widely used in scientific computing, data analysis, and machine learning.
-
Pandas: Pandas is a library for data manipulation and analysis in Python. It provides data structures and functions for handling and analyzing large datasets, such as CSV files or Excel spreadsheets. Pandas is widely used in data analysis, finance, and scientific research.
-
Django: Django is a web framework for building web applications in Python. It provides a set of tools and libraries for building web applications quickly and easily, including an ORM (Object-Relational Mapping) system for database access, a templating engine for rendering HTML pages, and a URL routing system for mapping URLs to views. Django is widely used in web development, and is particularly popular for building e-commerce sites and content management systems.
-
Flask: Flask is a lightweight web framework for building web applications in Python. It provides a minimal set of tools and libraries for building web applications, and is particularly well-suited for small projects or prototyping. Flask is widely used in web development, and is particularly popular for building APIs and microservices.
-
Pygame: Pygame is a library for building games in Python. It provides tools and functions for handling game graphics, input, and sound, as well as a set of game development tutorials and examples. Pygame is widely used in game development, and is particularly popular for building 2D games.
These are just a few examples of the many libraries and tools that the Python community develops and maintains. By using these libraries and tools, developers can extend the capabilities of Python and solve common problems more quickly and easily. The open-source nature of these libraries and tools also encourages collaboration and innovation within the Python community.
The Python community also organizes conferences, workshops, and meetups where developers can connect with each other in person. These events provide opportunities for developers to learn new skills, share their experiences, and network with other professionals in the industry.
Real-World Applications
Python is used extensively in a wide range of fields due to its versatility and ease of use. Some of the most popular fields where Python is used include data science, machine learning, artificial intelligence, web development, and scientific computing.
In data science, Python is a popular choice for tasks such as data analysis, data visualization, and data modeling. Libraries such as NumPy, Pandas, and Matplotlib provide a wide range of tools for working with large datasets and creating visualizations. Additionally, libraries such as Scikit-Learn and TensorFlow provide powerful machine learning tools for developing models quickly and efficiently.
Machine learning is another field where Python is widely used, thanks to libraries such as TensorFlow, PyTorch, and Scikit-Learn. These libraries provide a wide range of tools for neural network training, deep learning, and natural language processing. Python's simplicity and readability make it easy to develop and test machine learning models quickly and efficiently.
Python is also widely used in artificial intelligence (AI) applications such as chatbots, recommendation systems, and image recognition. Libraries such as Keras and TensorFlow provide powerful tools for developing deep learning models, while libraries such as OpenCV and Scikit-Image make it easy to work with images and videos. Python's simplicity and flexibility make it an ideal language for developing AI applications quickly and efficiently.
In web development, Python is used extensively with popular frameworks such as Django and Flask, which provide a range of tools for building web applications. Python's ease of use and flexibility make it easy to develop web applications quickly, while its large community of users and extensive libraries make it easy to find help and extend functionality.
Finally, Python is widely used in scientific computing for tasks such as simulations, data analysis, and visualization. Libraries such as NumPy, SciPy, and Matplotlib provide a wide range of tools for scientific computing, while libraries such as Pygame and VPython make it easy to create simulations and visualizations. Python's readability and flexibility make it an ideal language for scientific computing, where code needs to be both efficient and easy to understand.
Conclusion
In summary, Python is a high-level programming language known for its simplicity, versatility, and extensive standard library. It is widely used in various fields such as data science, machine learning, artificial intelligence, web development, and scientific computing. Python's demand in the job market continues to rise, making it a valuable skill for job seekers. Python's large and active community of developers provides support through various channels and creates a vast array of libraries and tools. Python's numerous real-world applications make it a reliable choice for developers in a wide range of industries. Overall, learning Python can open up new career opportunities, increase earning potential, and provide a foundation for developing a variety of useful applications.
References
- Python Software Foundation. (n.d.). About Python. Retrieved from https://www.python.org/about/
- Shanahan, D. (2021, March 23). The popularity of Python continues to rise. Towards Data Science. Retrieved from https://towardsdatascience.com/the-popularity-of-python-continues-to-rise-2021-edition-5d5b5aa3cc5c
- Stack Overflow. (2020). Stack Overflow Developer Survey 2020. Retrieved from https://insights.stackoverflow.com/survey/2020
- Python Software Foundation. (n.d.). Python Success Stories. Retrieved from https://www.python.org/success-stories/
- McKinney, W. (2017). Python for Data Analysis: Data Wrangling with Pandas, NumPy, and IPython. O'Reilly Media, Inc.
- TensorFlow. (n.d.). TensorFlow. Retrieved from https://www.tensorflow.org/
- PyTorch. (n.d.). PyTorch. Retrieved from https://pytorch.org/
- Scikit-Learn. (n.d.). Scikit-Learn. Retrieved from https://scikit-learn.org/stable/
- Keras. (n.d.). Keras. Retrieved from https://keras.io/
- OpenCV. (n.d.). OpenCV. Retrieved from https://opencv.org/
- Django. (n.d.). Django. Retrieved from https://www.djangoproject.com/
- Flask. (n.d.). Flask. Retrieved from https://flask.palletsprojects.com/en/2.1.x/
- NumPy. (n.d.). NumPy. Retrieved from https://numpy.org/
- SciPy. (n.d.). SciPy. Retrieved from https://www.scipy.org/
- Matplotlib. (n.d.). Matplotlib. Retrieved from https://matplotlib.org/
- Pygame. (n.d.). Pygame. Retrieved from https://www.pygame.org/news
- VPython. (n.d.). VPython. Retrieved from http://www.vpython.org/
- Rossum, G. V. (2009). Python 3000: the next generation. Retrieved from https://www.python.org/doc/essays/py3k-accounts/
- Zemlin, J. (2017, March 9). The road to Python 3.6: the early years. Opensource.com. Retrieved from https://opensource.com/article/17/3/python-36